Send to REST Service Action
Infiniti includes the ability to send form data to a REST service, via the "Send to REST Service" action. The action allows you to specify:
- Endpoint URL
- HTTP method (defaults to POST)
- Basic Auth username and password
- Custom Headers
- Access token
- Send as multi-part (true/false)
- Body document (JSON or XML)
- Output Path (JSONPath or XPath)
The action provides two outputs:
- Status (the HTTP response such as "OK", "CREATED")
- Response (the HTTP response body, if any)
- Output Path Data (the results, as a value or snippet, of the Output Path against the Response)
1. Basic Action configuration
The Send to REST Service action can be added to a project in Design, either on the Finish page or as part of a Workflow transition.
The input parameters for the action are as follows:
Input Name | Description | Required? |
---|---|---|
URL | The endpoint of the service | Yes |
HTTP Method | The HTTP method to use for the request. Supported values are "GET", "POST", "PUT", "DELETE" and "PATCH". Defaults to "POST" if not specified. | No |
Basic Auth Username | The username to supply for HTTP Basic Auth, if required by the service. | No |
Basic Auth Password | The password to supply for HTTP Basic Auth, if required by the service. | No |
Custom Headers | Any custom headers (such as an authorisation token or other special data) to supply as part of the request. Multiple headers can be specified if necessary. | No |
Send Access Token | True/false representing whether to send the user's SSO access token (from e.g. a SAML identity) as an Authorization header for the request. | No |
Send as Multipart | Whether to construct a multi-part request (for example, if the body document will exceed the service's maximum request length). | No |
Output Path | A parameter that defines a path in the REST response that will be output as the Output Path Data parameter OR a key/value pair that specifies a response header value to return. | No |
2. Sending a body document
The action accepts an optional body document to be sent as the content of the request. If required by the service, this should be included as a template within the project and attached as a Action Document.
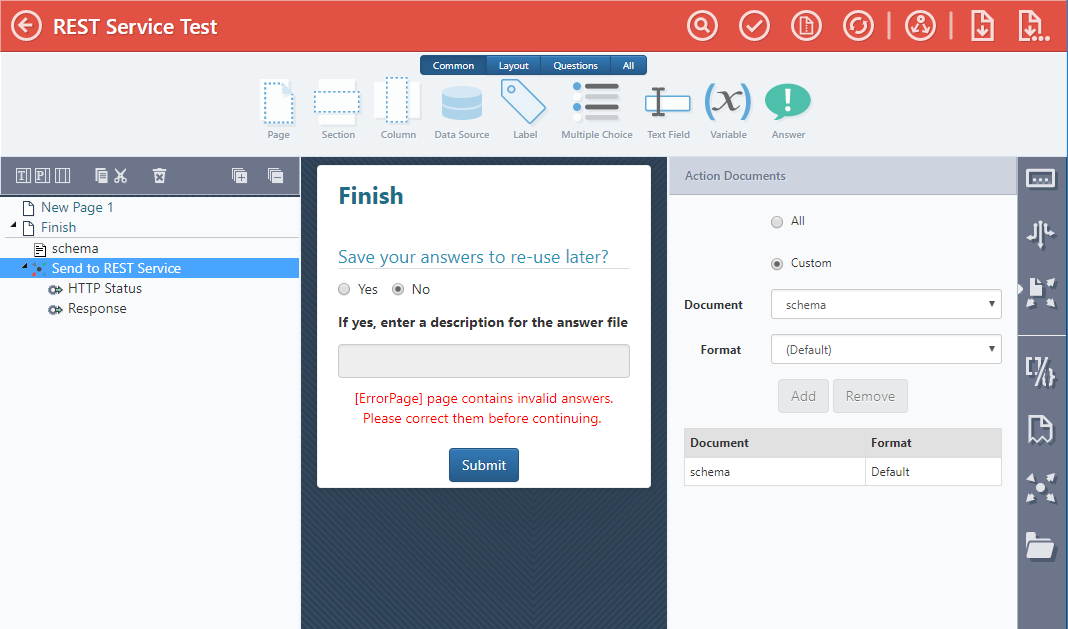
Selecting a body document for the REST request
Document type
The Action will automatically detect the type of document supplied (JSON or XML) and configure the request headers appropriately.
Document counts
Only a single JSON or XML body document can be supplied. If more than one is supplied, either explicitly through "Custom" action document settings, or implicitly through multiple templates being present in the project and not deactivated by conditions, the REST Service action will throw an error.
Attachments
If an attachment is to be included with the rest service, you can use two methods:
-
If your request body uses a JSON document then add the appropriate JSON placeholder onto the attachment answer - this will insert the uploaded/generated document into the JSON as base 64
-
Alternatively attach the document to the REST action and set the "Send as multipart" input
3. Custom headers
Custom headers, if required by the service, can be supplied using the Custom Header action parameter. Zero or more headers may be specified as a key-value pair, and will be sent as part of the REST request.
4. Using the Status and Response outputs
The Status and Response outputs can be referenced by subsequent Actions, for example to drive the display of a message to the user, or to store the response body in a database for later reference.
5. Using an Output Path and the Output Path Data
A path to a value or node in the Response to the REST call can be specified using the Output Path parameter.
Possible path formats are JSONPath for JSON responses or XPath for XML responses.
Alternatively a key/value pair with the key of header can be specified to return the value of that header from the REST call response.
JSONPath
JSONPath expressions always refer to a JSON structure in the same way as XPath expression are used in combination with an XML document. Since a JSON structure is usually anonymous and doesn't necessarily have a "root member object" JSONPath assumes the abstract name $ assigned to the outer level object.
JSONPath expressions in Infiniti use the dot–notation (JSONPath uses a 0 based index):
$.store.book[0].title
An example Output Path Data value in this case might be "The Da Vinci Code"
or if the Response only returns a single node you can leave out the index.
$.store.book.title
If you use a non indexed call (like the one just above) against an array or a response with many nodes you will get all the relevant title values as a | separated string
An example Output Path Data value in this case might be "The Da Vinci Code|The Lord of the Rings|Anne Frank Diary of a Young Girl"
If you call a path that is an node rather than a value, you will get a JSON snippet in return.
For example an Output Path of:
$.address
Might result in a JSON snippet like this as the Output Path Data value, because the path does not have a simple value.
{ "street": "Kulas Light", "suite": "Apt. 556", "city": "Gwenborough", "zipcode": "92998-3874", "geo": { "lat": "-37.3159", "lng": "81.1496" } }
XPath
XPath uses a 1 based index.
/bookstore/book[1]
An example Output Path Data value in this case might be "The Da Vinci Code"
or if the Response only returns a single node you can leave out the index.
/bookstore/book
If you use a non indexed call (like the one just above) against an array or a response with many nodes you will get all the relevant title values as a | separated string
An example Output Path Data value in this case might be "The Da Vinci Code|The Lord of the Rings|Anne Frank Diary of a Young Girl"
If you call a path that is an node rather than a value, you will get an XML snippet in return.
For example an Output Path of:
/CUSTOMER
Might result in an XML snippet like this as the Output Path Data value, because the path does not have a simple value.
<ID>18</ID><FIRSTNAME>Sylvia</FIRSTNAME><LASTNAME>Fuller</LASTNAME><STREET>158 - 20th Ave.</STREET><CITY>Paris</CITY>
Header
This is used to access a header value from the REST call response. Generally you would be retrieving the value of a custom header returned with the response, but standard headers can be retrieved as well.
Only a single header's value can be retrieved and you cannot retrieve a header as well as a JSONPath or XmlPath.
You specify the key/value pair as follows:
header=[header name]
For example
header=Content-Type
and this will return the string value of that header in the REST response. In the example above this would look something like:
application/json; charset=utf-8
6. Sample project
The following section will walk through constructing a project to send some REST data to the https://jsonplaceholder.typicode.com/ test service.
Sample JSON schema
{
"$schema": "http://json-schema.org/draft-04/schema#",
"definitions": {},
"id": "http://example.com/example.json",
"properties": {
"body": {
"description": "An explanation about the purpose of this instance.",
"id": "/properties/body",
"type": "string"
},
"title": {
"description": "An explanation about the purpose of this instance.",
"id": "/properties/title",
"type": "string"
},
"userId": {
"description": "An explanation about the purpose of this instance.",
"id": "/properties/userId",
"type": "integer"
}
},
"type": "object"
}
Project setup
- Create a new project in Design
- Import the
example.schema.json
document as a project template - Create some Text Input questions and map their Answers to the template placeholders
- Add a "Send to REST Service" action to the project's Finish page
- Supply parameters for the action as follows:
- URL: https://jsonplaceholder.typicode.com/posts
- HTTP Method: POST
- Optionally,
- EITHER: add an Output Path value of
$[4].title
to get an Output Path Data value which should match the title of the 5th post in the Response. - OR: add an Output Path value of
header=CF-RAY
to get an Output Path Data value which will match the value of the header CF-RAY in the Response.
- Optionally, set up the Action Documents to pass only the correct document to the Action. If there are no other JSON or XML templates in the project this step is unnecessary, as the default "All" setting will work fine
- For Delete of the resource created in Step 5 supply following parameters to the action with no action document
- URL: https://jsonplaceholder.typicode.com/posts/{UserIdCreated}
- HTTP Method: DELETE
Troubleshooting Modes
To troubleshoot the problems in Send to REST Service action, make sure to check Troubleshooting Mode in the project Publish Options.
Send to REST Service Action Troubleshooting
To log more detailed troubleshooting data including all REST parameters and values, check Show Variables and Show Invisible Datasources. Also in AppSettings.json file under produce, make sure to add "ShowDatasourceTroubleshootingErrors": "True" under AppSettings.
"AppSettings": {
"ShowDatasourceTroubleshootingErrors": "True"
},
Updated 24 days ago
Take a look at the REST Data Source type, to retrieve data posted in a previous form session.